Hello, I have read some of the solution replied in discussion about the error on creating .rtg files. My problem is that, I created my own roads in GIS editor and then try to create a .rtg in routing explorer but the result is an error saying "object reference not set to an instance of the object". What should be the solution about this??
Creating .rtg Error
Hi Joseph,
Routing explorer can only be used to handle the data under DecimalDegree MapUnit, and it cannot deal with OneWay data.
So if your data require these features, please try to build RTG file like this:
RtgRoutingSource.GenerateRoutingData(rtgShapeFilePath, sourceShapeFilePath, routableShapeFilePath, BuildRoutingDataMode.Rebuild, GeographyUnit.Meter, DistanceUnit.Feet);
Attached is an utility for build your RTG file, you should want to did some changes for make it works for you.
Regards,
Don
BuildingOnewayRoadRoutingData.zip (13.4 KB)
Hello, I am aware about the comments in the file you sent me. I just add those needed references and run it but an error occur maybe because I need to edit some of the code but I don’t know where to start. I tried to analyze the codes but I’m afraid to change it to a worse result. Would you help me debug the codes. Since what only matters to me is to used the roads I have made in GIS editor for routing and I need a .rtg file for it,right? Here are the codes and the error:
using
System;
using
System.Collections.ObjectModel;
using
System.Windows.Forms;
using
ThinkGeo.MapSuite.Core;
using
ThinkGeo.MapSuite.Routing;
using
System.IO;
namespace
BuildingOnewayRoadRoutingData
{
public
partial
class
Form1 : Form
{
private
string
sourceShapeFilePath;
private
string
routableShapeFilePath;
private
string
rtgShapeFilePath;
private
FeatureSource routableShapeFileFeatureSource;
public
Form1()
{
InitializeComponent();
sourceShapeFilePath = txtSourceShpFilePath.Text;
routableShapeFilePath = txtRoutableShpFile.Text;
rtgShapeFilePath = Path.ChangeExtension(routableShapeFilePath,
“rtg”
);
}
private
void
btnGenerateRoutableShapeFile_Click(
object
sender, EventArgs e)
{
ShapeFileFeatureSource originalFeatureSource =
new
ShapeFileFeatureSource(sourceShapeFilePath);
// Todo: Set progress bar, can be removed if you would like
originalFeatureSource.Open();
buildingPgbar.Maximum = originalFeatureSource.GetCount();
originalFeatureSource.Close();
buildingPgbar.Value = 0;
buildingPgbar.Visible =
true
;
btnGenerateRoutableShapeFile.Enabled =
false
;
RtgRoutingSource.GeneratingRoutableShapeFile +=
new
EventHandler<GeneratingRoutableShapeFileRoutingSourceEventArgs>(RtgRoutingSource_GeneratingRoutableShapeFile);
RtgRoutingSource.GenerateRoutableShapeFile(sourceShapeFilePath, routableShapeFilePath, OverwriteMode.Overwrite);
btnGenerateRoutableShapeFile.Enabled =
true
;
MessageBox.Show(
“Routable Shape File has been Build! You can Start building routing index with it now.”
);
}
private
void
RtgRoutingSource_GeneratingRoutableShapeFile(
object
sender, GeneratingRoutableShapeFileRoutingSourceEventArgs e)
{
if
(buildingPgbar.Value < buildingPgbar.Maximum)
{
buildingPgbar.Value += 1;
}
Application.DoEvents();
}
private
void
btnBuildRoutingData_Click(
object
sender, EventArgs e)
{
routableShapeFileFeatureSource =
new
ShapeFileFeatureSource(routableShapeFilePath);
routableShapeFileFeatureSource.Open();
buildingPgbar.Maximum = routableShapeFileFeatureSource.GetCount();
buildingPgbar.Value = 0;
buildingPgbar.Visible =
true
;
btnBuildRoutingData.Enabled =
false
;
RtgRoutingSource.BuildingRoutingData +=
new
EventHandler<BuildingRoutingDataRtgRoutingSourceEventArgs>(RtgRoutingSource_BuildingRoutingData);
RtgRoutingSource.GenerateRoutingData(rtgShapeFilePath, sourceShapeFilePath, routableShapeFilePath, BuildRoutingDataMode.Rebuild, GeographyUnit.Meter, DistanceUnit.Feet);
btnBuildRoutingData.Enabled =
true
;
MessageBox.Show(
“Completed”
);
}
void
RtgRoutingSource_BuildingRoutingData(
object
sender, BuildingRoutingDataRtgRoutingSourceEventArgs e)
{
if
(buildingPgbar.Value < buildingPgbar.Maximum)
{
buildingPgbar.Value += 1;
}
Application.DoEvents();
if
(!routableShapeFileFeatureSource.IsOpen)
{
routableShapeFileFeatureSource.Open();
}
Feature routableFeature = routableShapeFileFeatureSource.GetFeatureById(e.RouteSegment.FeatureId,
new
Collection<
string
>() {
“ONE_WAY”
});
LineShape lineShape = GetLineShapeFromBaseShape(routableFeature);
// If it’s one-way road
if
(routableFeature.ColumnValues[
“ONE_WAY”
].ToString() ==
“1”
)
{
e.RouteSegment.StartPointAdjacentIds.Clear();
}
// analysis adjacent one-way road features
Collection<
string
> removedStartPointAdjacentIds = GetRemovedAdjacentIds(e.RouteSegment.StartPointAdjacentIds,
new
PointShape(lineShape.Vertices[0]));
foreach
(
string
id
in
removedStartPointAdjacentIds)
{
e.RouteSegment.StartPointAdjacentIds.Remove(id);
}
Collection<
string
> removedEndPointAdjacentIds = GetRemovedAdjacentIds(e.RouteSegment.EndPointAdjacentIds,
new
PointShape(lineShape.Vertices[lineShape.Vertices.Count - 1]));
foreach
(
string
id
in
removedEndPointAdjacentIds)
{
e.RouteSegment.EndPointAdjacentIds.Remove(id);
}
// Todo: currently we haven’t process it, because we are unable to change the Huristic Value.
// but we can uncomment below code if we are using the Dikjistra algorithm
// e.RouteSegment.Weight /= (double)routableFeature.ColumnValues[“MPH”];
}
private
Collection<
string
> GetRemovedAdjacentIds(Collection<
string
> adjacentIds, PointShape intersectingPoint)
{
Collection<
string
> removedIds =
new
Collection<
string
>();
foreach
(
string
id
in
adjacentIds)
{
Feature adjacentFeature = routableShapeFileFeatureSource.GetFeatureById(id,
new
Collection<
string
>() {
“ONE_WAY”
});
if
(adjacentFeature.ColumnValues[
“ONE_WAY”
].ToString() ==
“1”
)
{
LineShape adjacentLineShape = GetLineShapeFromBaseShape(adjacentFeature);
double
distanceFromAdjacentStartToIntersecting =
new
PointShape(adjacentLineShape.Vertices[0]).GetDistanceTo(intersectingPoint, GeographyUnit.Feet, DistanceUnit.Meter);
double
distanceFromAdjacentEndToIntersecting =
new
PointShape(adjacentLineShape.Vertices[adjacentLineShape.Vertices.Count - 1]).GetDistanceTo(intersectingPoint, GeographyUnit.Feet, DistanceUnit.Meter);
if
(distanceFromAdjacentEndToIntersecting < distanceFromAdjacentStartToIntersecting)
{
removedIds.Add(id);
}
else
if
(distanceFromAdjacentEndToIntersecting < distanceFromAdjacentStartToIntersecting)
{
removedIds.Add(id);
}
}
}
return
removedIds;
}
private
static
LineShape GetLineShapeFromBaseShape(Feature lineFeature)
{
BaseShape baseShape = lineFeature.GetShape();
LineShape lineShape = baseShape
as
LineShape;
if
(lineShape ==
null
)
{
MultilineShape lineShapes = ((MultilineShape)baseShape);
Collection<Vertex> vertices =
new
Collection<Vertex>();
foreach
(LineShape line
in
lineShapes.Lines)
{
for
(
int
i = 0; i < line.Vertices.Count; i++)
{
vertices.Add(line.Vertices<i>);
}
}
lineShape =
new
LineShape(vertices);
lineShape.Id = baseShape.Id;
lineShape.Tag = baseShape.Tag;
}
return
lineShape;
}
private
void
btnRoutableShpFile_Click(
object
sender, EventArgs e)
{
OpenFileDialog fd =
new
OpenFileDialog();
fd.Filter =
“Shape Files (*.shp)|*.shp”
;
if
(fd.ShowDialog() == DialogResult.OK)
{
txtRoutableShpFile.Text = fd.FileName;
}
}
private
void
btnSourceShpBrowse_Click(
object
sender, EventArgs e)
{
OpenFileDialog fd =
new
OpenFileDialog();
fd.Filter =
“Shape Files (*.shp)|*.shp”
;
if
(fd.ShowDialog() == DialogResult.OK)
{
txtSourceShpFilePath.Text = fd.FileName;
}
}
}
}
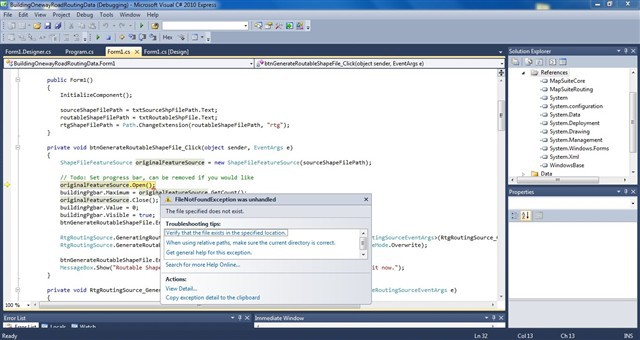
Hi Joseph,
Sorry I haven’t noticed your update here.
Have you fixed this problem? It looks you have another exception in today’s new topic.
Regards,
Don
Not yet but I already sent you the ShapeFile in other Topic I created!
Hi Joseph,
I will reply you on post thinkgeo.com/forums/MapSuite/tabid/143/aft/11858/Default.aspx , would you please check it there?
Best Regards
Summer