Arber,
Instead of a custom Style, I think you need to create a Custom CurvedLineShape which can Save To / Load from a PostGre WKT. Here is a simple example how to do it by creating a new shape from BaseShape. Just call LoadFromCustomWellKnownData to load the special WKT from database, and call GetCustomWellKnownText() and save it. It should work for you.
// The CurvedLineShape Class
class CurvedLineShape : BaseShape
{
private PointShape centerPoint;
private PointShape startPoint;
private double angle;
public CurvedLineShape()
: this(new PointShape(0, 0), new PointShape(0, 0), 0)
{ }
public CurvedLineShape(PointShape centerPoint, PointShape startPoint, int angle)
{
this.centerPoint = centerPoint;
this.startPoint = startPoint;
this.angle = angle;
}
public CurvedLineShape(string wellKnownText)
{
if (wellKnownText == null || !wellKnownText.StartsWith("CurvedLineShape"))
{
throw new ArgumentException("The wellKnownText is not supported");
}
LoadFromCustomWellKnownData(wellKnownText);
}
protected override string GetWellKnownTextCore()
{
LineShape line = new LineShape();
line.Vertices.Add(new Vertex(startPoint));
double radius = Math.Sqrt(Math.Pow(startPoint.X - centerPoint.X, 2) + Math.Pow(startPoint.Y - centerPoint.Y, 2));
double startAngle = Math.Asin(startPoint.Y / radius);
for (int i = 1; i <= angle; i++)
{
double x = Math.Cos(Math.PI / 180 * i + startAngle) * radius + centerPoint.X;
double y = Math.Sin(Math.PI / 180 * i + startAngle) * radius + centerPoint.Y;
line.Vertices.Add(new Vertex(x, y));
}
return line.GetWellKnownText();
}
public string GetCustomWellKnownText()
{
StringBuilder sb = new StringBuilder();
sb.Append("CurvedLineShape(");
sb.Append(centerPoint.X + " " + centerPoint.Y);
sb.Append(",");
sb.Append(startPoint.X + " " + startPoint.Y);
sb.Append(",");
sb.Append(angle);
sb.Append(")");
return sb.ToString();
}
public void LoadFromCustomWellKnownData(string wellKnownText)
{
string wkt = wellKnownText.Replace("CurvedLineShape(", "");
wkt = wkt.Remove(wkt.Length - 1);
string[] wkts = wkt.Split(',');
string[] data = wkts[0].Split(' ');
this.centerPoint = new PointShape(Double.Parse(data[0]), Double.Parse(data[1]));
data = wkts[1].Split(' ');
this.startPoint = new PointShape(Double.Parse(data[0]), Double.Parse(data[1]));
this.angle = Double.Parse(wkts[2]);
}
protected override void LoadFromWellKnownDataCore(string wellKnownText)
{
}
}
// The references.
private void WpfMap_Loaded(object sender, RoutedEventArgs e)
{
InMemoryFeatureLayer inm = new InMemoryFeatureLayer();
inm.InternalFeatures.Add(new CurvedLineShape("CurvedLineShape(0 0,10 10, 90)").GetFeature());
inm.ZoomLevelSet.ZoomLevel01.DefaultLineStyle = LineStyles.Canal1;
inm.ZoomLevelSet.ZoomLevel01.ApplyUntilZoomLevel = ApplyUntilZoomLevel.Level20;
LayerOverlay overlay = new LayerOverlay();
overlay.Layers.Add(inm);
Map1.MapUnit = GeographyUnit.DecimalDegree;
Map1.CurrentExtent = new RectangleShape(-155.733, 95.60, 104.42, -81.9);
Map1.Overlays.Add(overlay);
Map1.Refresh();
}
Here is what looks like:
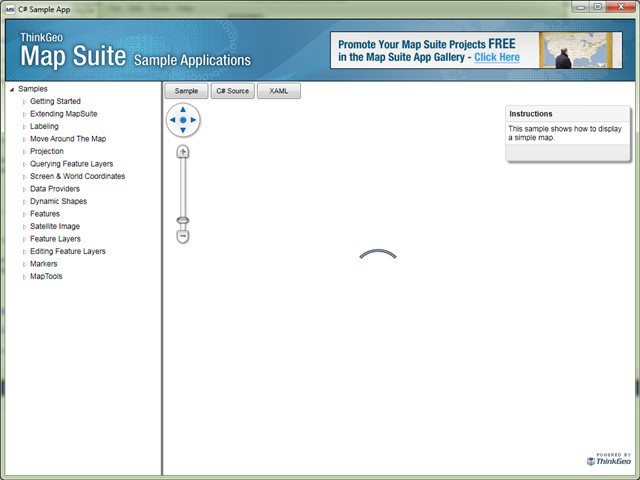
Thanks,
Bai